QUICK SUMMARY: This tutorial shows you how to build simple image recognition (aka binary image classifier) in pure Java. It will help you to get started with modern AI development using your Java skills. The resulting deep learning model will have the capability to recognize food type in a given image.
video below from TV Show Sillicon Valley to get the basic idea.
Image classifier uses deep learning to learn from examples how to automatically put images into appropriate categories / folders. In this tutorial we’ll use example images of food to build classifier that is able to distinguish hot-dog from not-hot-dog images. Watch the funnyStart by cloning this GitHub repo: https://github.com/deepnetts/hotdog-nothotdog
In order to run the example you need to download and install Deep Netts.
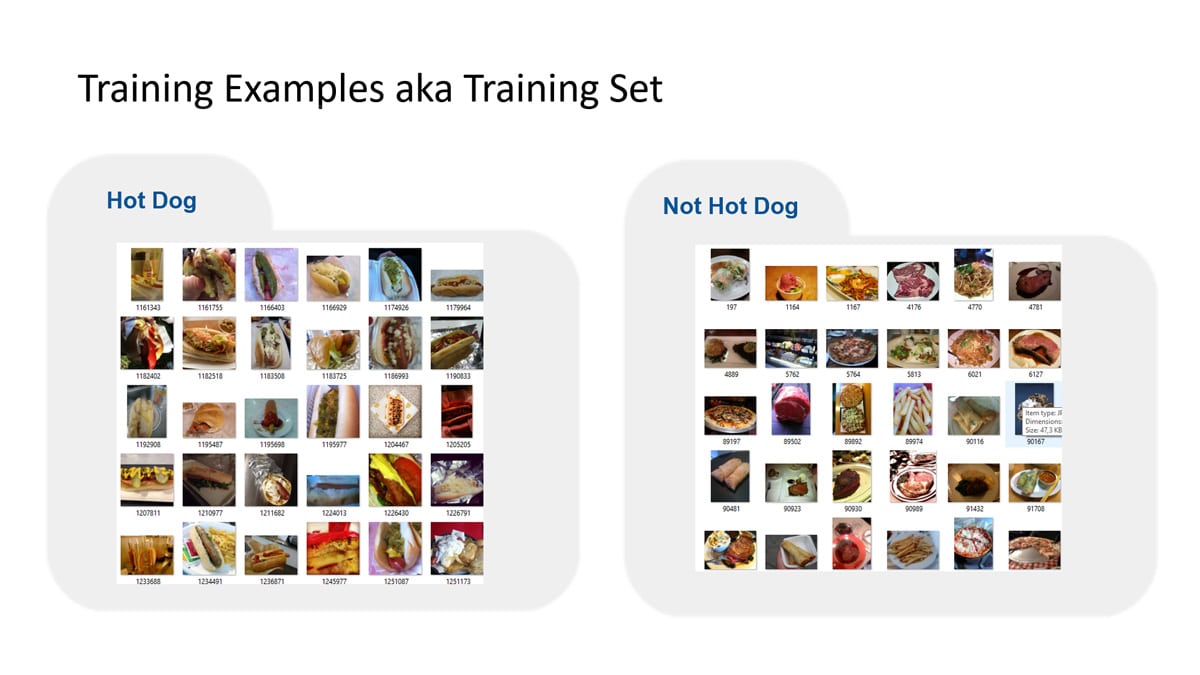
The following code loads images from a file system, and prepares them for neural network training.
ImageSet imageSet = new ImageSet(imageWidth, imageHeight, dataSetPath);
imageSet.setResizeStrategy(ImageResize.STRATCH);
imageSet.setInvertImages(true);
imageSet.zeroMean();
Convolutional neural networks are specialized for image recognition tasks, and the following code creates small convolutional network which is configured to perform binary image classification.
ConvolutionalNetwork convNet = ConvolutionalNetwork.builder()
.addInputLayer(imageWidth, imageHeight, 3)
.addConvolutionalLayer(3, Filter.ofSize(3))
.addMaxPoolingLayer(2, 2)
.addFullyConnectedLayer(32)
.addOutputLayer(1, ActivationType.SIGMOID)
.hiddenActivationFunction(ActivationType.LEAKY_RELU)
.lossFunction(LossType.CROSS_ENTROPY)
.build();
To train neural network using example/training images you need to run the backpropagation training algorithm using loaded image set using the following code:
BackpropagationTrainer trainer = convNet.getTrainer();
trainer.setStopError(0.03f)
.setLearningRate(0.01f)
.setStopAccuracy(0.97f)
.setStopEpochs(300);
trainer.train(imageSet);
To see how accurately neural network is able to recognize images perform the following test:
EvaluationMetrics testResults = convNet.test(imageSet);
System.out.println(testResults);
To save a trained neural network into file, to be able to use it in your app just call the save method and specify name of the file to save the network into:
// SAVE THE MODEL - save trained neural network to file
convNet.save("HotDogClassifier.dnet");
To use the trained image classifier in your app use the following code snippet:
// load image from file
BufferedImage image = ImageIO.read(new File("hotdog-dataset/hot_dog/7896.jpg")).getSubimage(0, 0, 64, 64);
// load a trained convolutional network
ConvolutionalNetwork convNet = ConvolutionalNetwork.load("HotDogClassifier.dnet", ConvolutionalNetwork.class);
// instantiate an image classifier using convolutional neural network
ImageClassifier<BufferedImage> imageClassifier = new ImageClassifierNetwork(convNet);
// classify image and get results
Map<String, Float> results = imageClassifier.classify(image); // result is a map with image labels as keys and coresponding probability
Required Resources
In order to run examples from this tutorial you need the following:
- Deep Netts deep learning Java library & Visual AI Builder tool download
- Step-by-step installation tutorial is available in Quick Start tutorial
- Full source code available on GitHub: https://github.com/deepnetts/hotdog-nothotdog